FiniteLattice
Elements
FiniteLattice are a particular case of poset. Their elements have the following properties:
For all \(x, y\) of a poset, there exists a unique infimum and a unique supremum:
PrimeFactors example
[1]:
from galactic.algebras.examples.arithmetic import PrimeFactors
[2]:
PrimeFactors(24) & PrimeFactors(18)
[2]:
[3]:
PrimeFactors(24) | PrimeFactors(18)
[3]:
Color example
[4]:
from galactic.algebras.examples.color import Color
[5]:
color1 = Color(red=0.8, green=0.5, blue=0.7)
color1
[5]:
[6]:
color2 = Color(red=0.5, green=1.0, blue=0.9)
color2
[6]:
[7]:
color1 & color2
[7]:
[8]:
color1 | color2
[8]:
Collections
All operations on poset are available and some extras features:
get the minimum and maximum elements from a lattice;
get the atoms and the co-atoms elements from a lattice;
get the meet-irreducible and the join-irreducible elements from a lattice;
get the meet-irreducible generators and the join-irreducible generators of an element of the lattice.
PrimeFactors example
[9]:
from galactic.algebras.lattice import FiniteLattice, ReducedContextDiagram
from galactic.algebras.poset import HasseDiagram
from galactic.algebras.examples.arithmetic import PrimeFactorsRenderer
[10]:
integers = [
PrimeFactors(2),
PrimeFactors(3),
PrimeFactors(5),
PrimeFactors(7),
PrimeFactors(9),
]
lattice = FiniteLattice[PrimeFactors](domain=integers)
HasseDiagram[PrimeFactors](
lattice,
domain_renderer=PrimeFactorsRenderer(
meet_semi_lattice=True,
join_semi_lattice=True,
),
)
[10]:
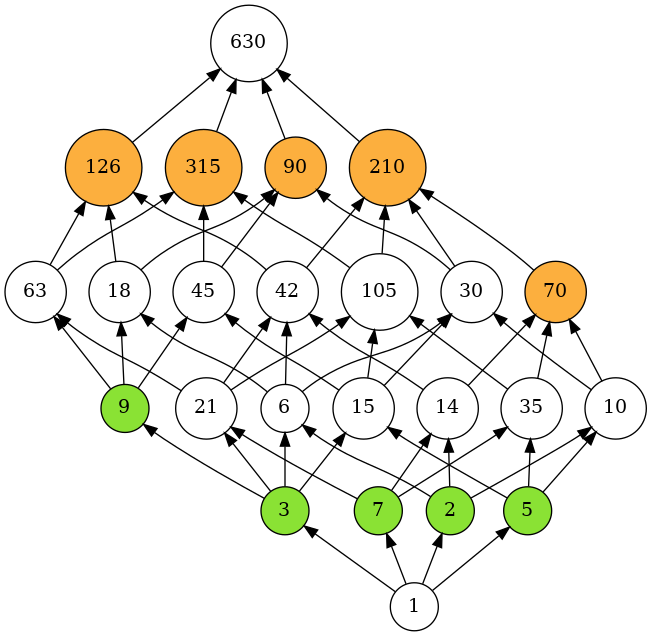
[11]:
lattice.minimum
[11]:
[12]:
lattice.maximum
[12]:
[13]:
display(*lattice.atoms)
[14]:
display(*lattice.co_atoms)
[15]:
display(*lattice.meet_irreducible)
[16]:
display(*lattice.join_irreducible)
[17]:
display(*lattice.smallest_meet_irreducible(PrimeFactors(30)))
[18]:
display(*lattice.greatest_join_irreducible(PrimeFactors(30)))
Color example
[19]:
from galactic.algebras.examples.color import Color, ColorRenderer
from galactic.algebras.lattice import FiniteLattice
[20]:
color3 = Color(red=1.0, green=0.7, blue=0.5)
color3
[20]:
[21]:
color4 = Color(red=0.6, green=1.0, blue=0.1)
color4
[21]:
[22]:
color5 = Color(red=0.4, green=0.6, blue=0.7)
color5
[22]:
[23]:
lattice = FiniteLattice[Color](
domain=[
color3,
color4,
color5,
]
)
[24]:
HasseDiagram[Color](lattice, domain_renderer=ColorRenderer())
[24]:
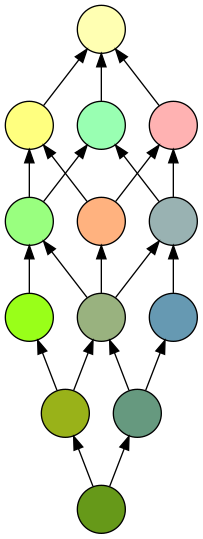
[25]:
lattice.extend([color1])
[26]:
HasseDiagram[Color](lattice, domain_renderer=ColorRenderer())
[26]:
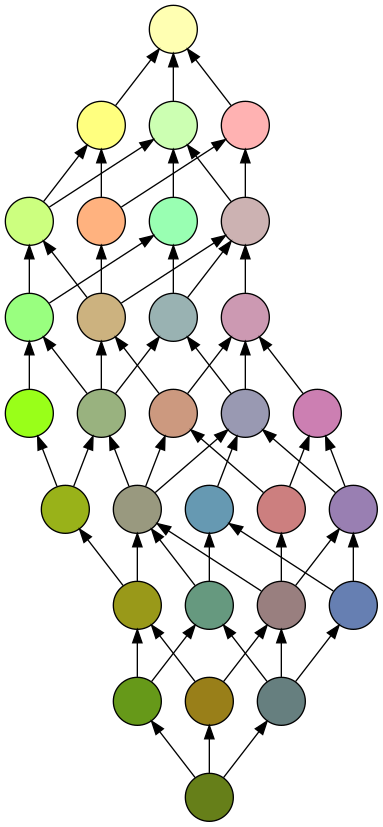
[27]:
ReducedContextDiagram(
lattice,
domain_renderer=ColorRenderer(),
co_domain_renderer=ColorRenderer(),
)
[27]:
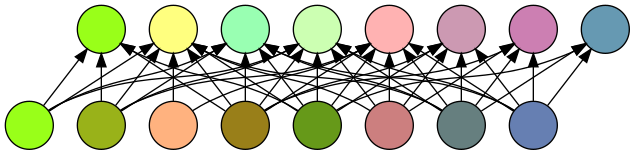
Context
There exists a bijection between a lattice and its minimal binary table called its equivalent context:
the rows are composed by the meet-irreducible
the columns are composed by the join-irreducible
the boolean value for row \(i\) and column \(j\) is True if \(i\geq j\)
PrimeFactors example
[28]:
integers = [
PrimeFactors(2),
PrimeFactors(3),
PrimeFactors(5),
PrimeFactors(7),
PrimeFactors(9),
]
lattice = FiniteLattice[PrimeFactors](domain=integers)
ReducedContextDiagram(
lattice,
domain_renderer=PrimeFactorsRenderer(join_irreducible=True),
co_domain_renderer=PrimeFactorsRenderer(meet_irreducible=True),
)
[28]:
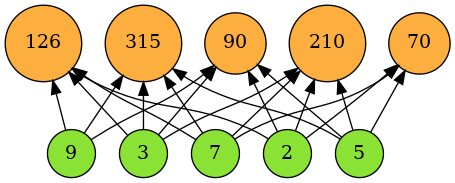
[29]:
from galactic.algebras.relational import BinaryTable
BinaryTable(
lattice.reduced_context,
domain_renderer=PrimeFactorsRenderer(join_irreducible=True),
co_domain_renderer=PrimeFactorsRenderer(meet_irreducible=True),
)
[29]:
126 |
315 |
210 |
90 |
70 |
|
---|---|---|---|---|---|
\(2=2\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
|
\(7=7\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
|
\(5=5\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
|
\(3=3\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
|
\(9=3^2\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |