Concept lattice
A population can be created using a collection of python objects:
[1]:
from galactic.population import Population
individuals = {48: 48, 36: 36, 64: 64, 56: 56, 84: 84}
population = Population(individuals)
population
[1]:
<galactic.population.Population at 0x7f217c1a2d90>
[2]:
list(population)
[2]:
['48', '36', '64', '56', '84']
A lattice can be created from a population using a set of strategies:
[3]:
from galactic.algebras.poset import HasseDiagram, IterativeDiagram
from galactic.concepts import (
ConceptRenderer,
HierarchicalRenderer,
ConceptLatticeRenderer,
ConceptLattice,
ConceptClosure,
Concept,
)
from galactic.examples.arithmetic import (
MultipleDescription,
DivisorDescription,
Integer,
)
characteristic = Integer()
descriptions = [MultipleDescription(characteristic), DivisorDescription(characteristic)]
closure = ConceptClosure(population, descriptions)
concept = Concept(closure)
concept
[3]:
Predicates:
M(4)
D(4032)
Individuals:
48
36
64
56
84
[4]:
from galactic.examples.arithmetic import MultipleStrategy, DivisorStrategy
lattice = ConceptLattice.create(
population, descriptions, [DivisorStrategy(characteristic)]
)
[5]:
lattice.global_entropy
[5]:
4.077819531114783
[6]:
lattice.global_stability
[6]:
0.28125
[7]:
lattice.global_logarithmic_stability
[7]:
0.05928469016817753
[8]:
lattice.global_information
[8]:
20.389097655573917
[9]:
list(str(concept) for concept in lattice.domain)
[9]:
['M(4) and D(4032)',
'M(4) and D(1008)',
'M(4) and D(1344)',
'M(4) and D(576)',
'M(4) and D(504)',
'M(4) and D(336)',
'M(12) and D(144)',
'M(8) and D(448)',
'M(16) and D(192)',
'M(12) and D(252)',
'M(28) and D(168)',
'M(48) and D(48)',
'M(36) and D(36)',
'M(64) and D(64)',
'M(56) and D(56)',
'M(84) and D(84)',
'M(0) and D(1)']
[10]:
for diagram in IterativeDiagram(
lattice,
graph_renderer=ConceptLatticeRenderer(),
domain_renderer=ConceptRenderer(),
edge_renderer=HierarchicalRenderer(),
):
display(diagram)

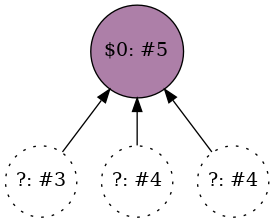
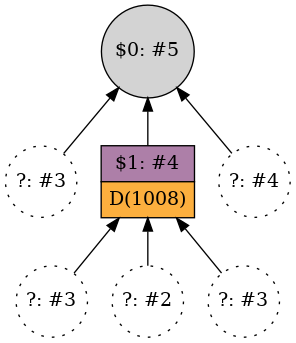
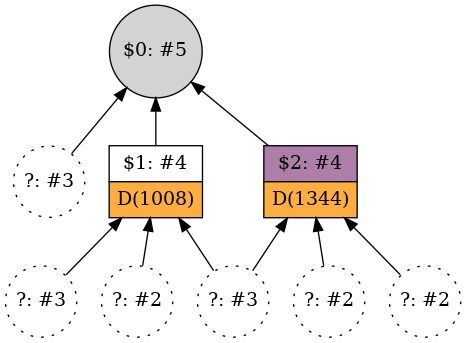
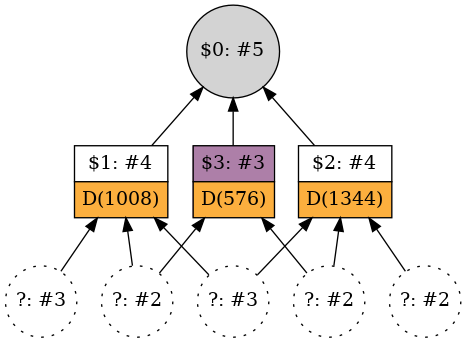
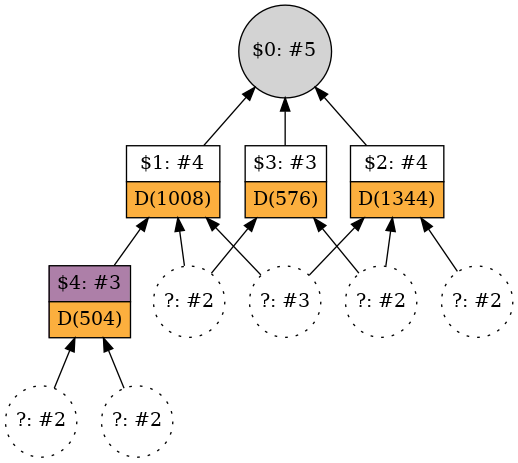
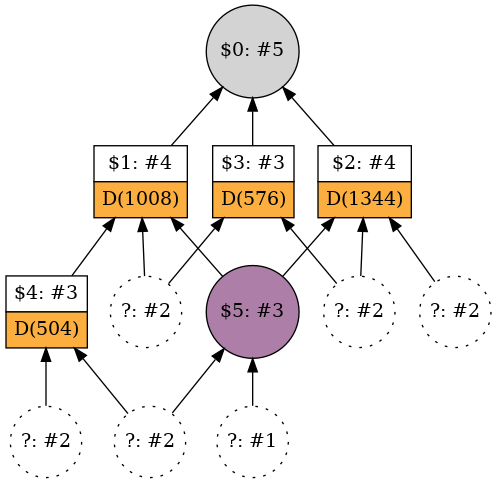
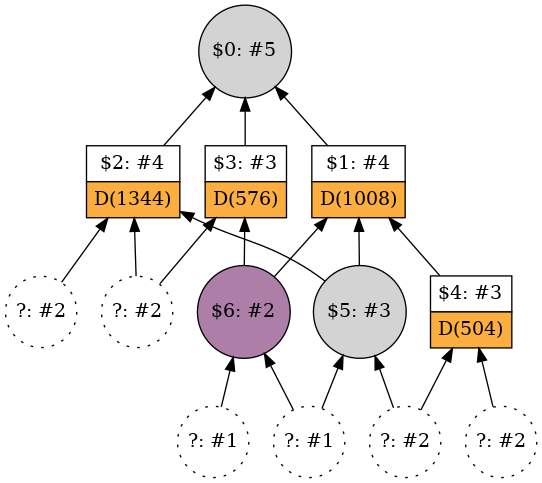
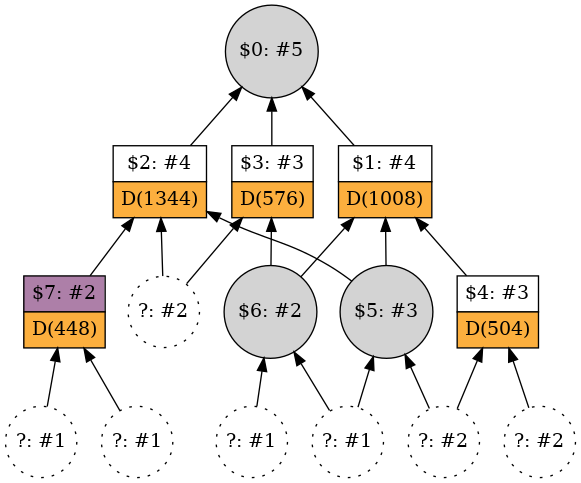
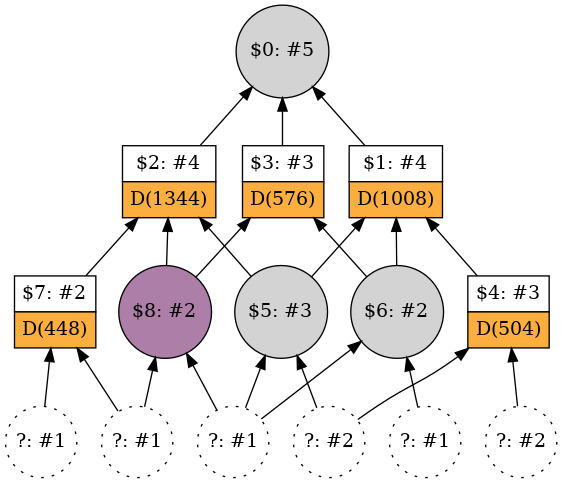
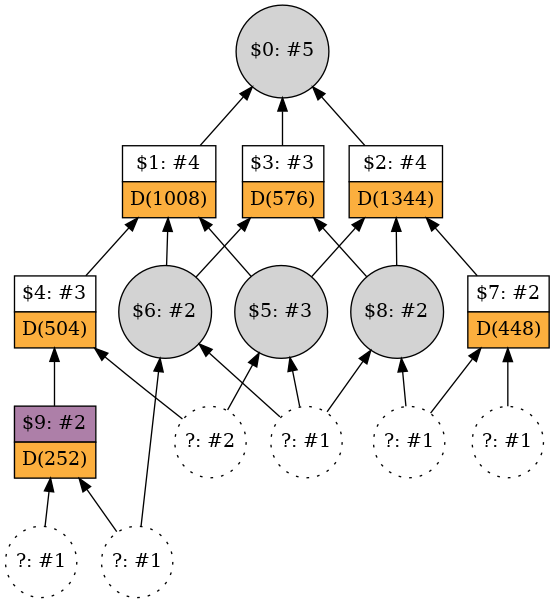
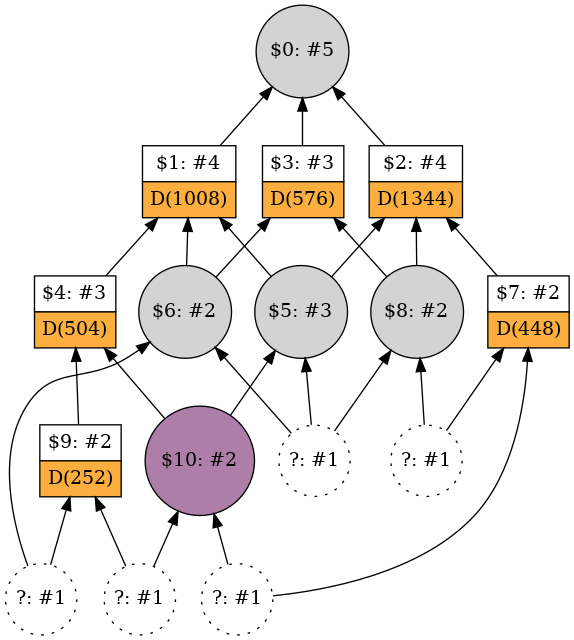
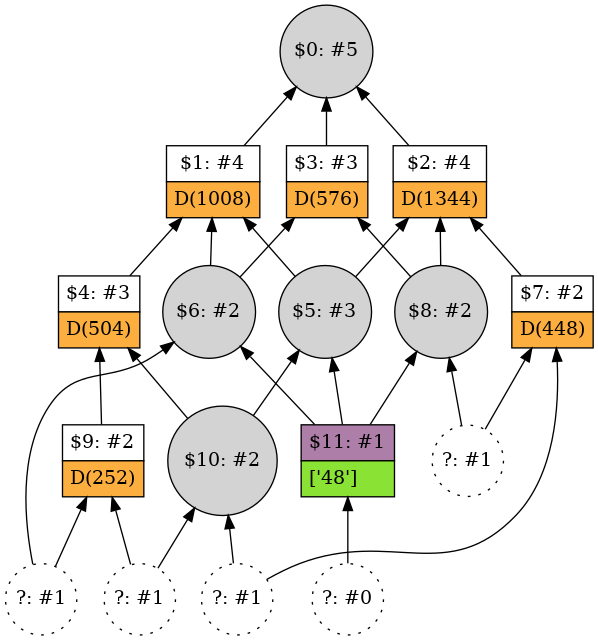
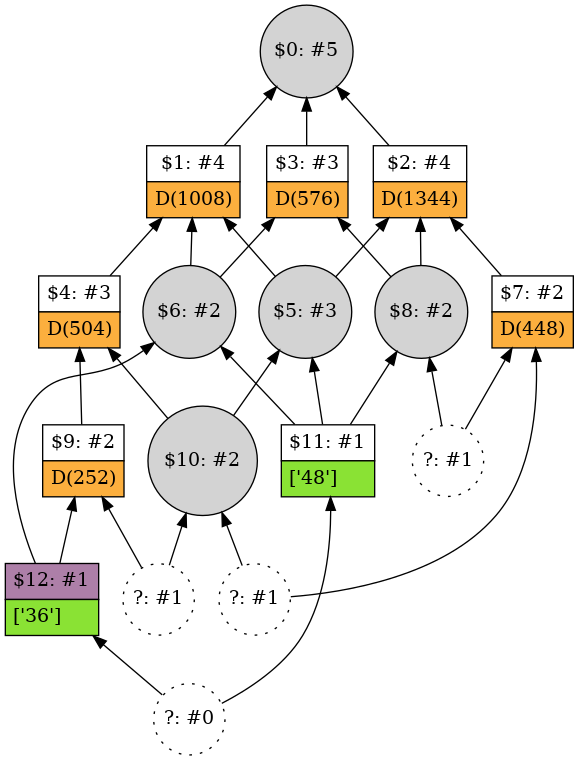
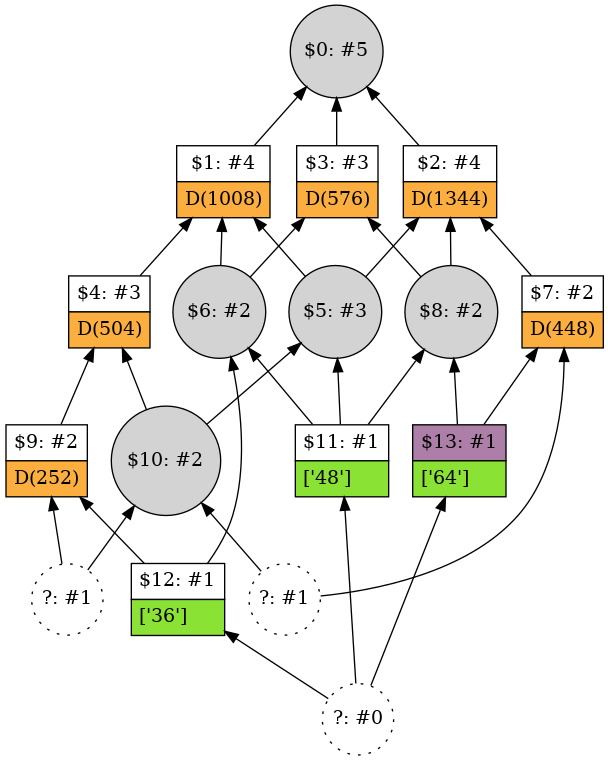
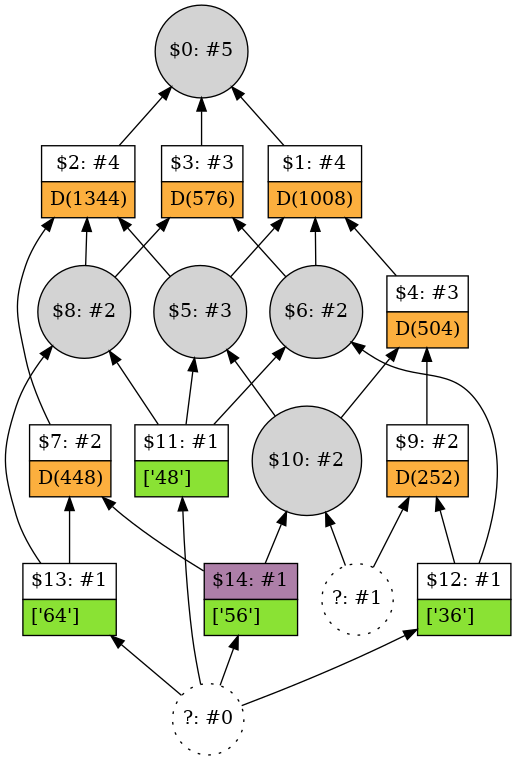
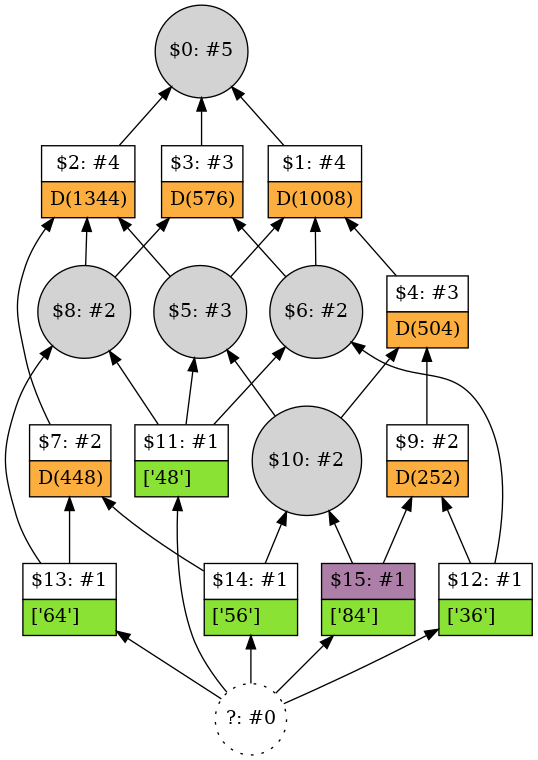
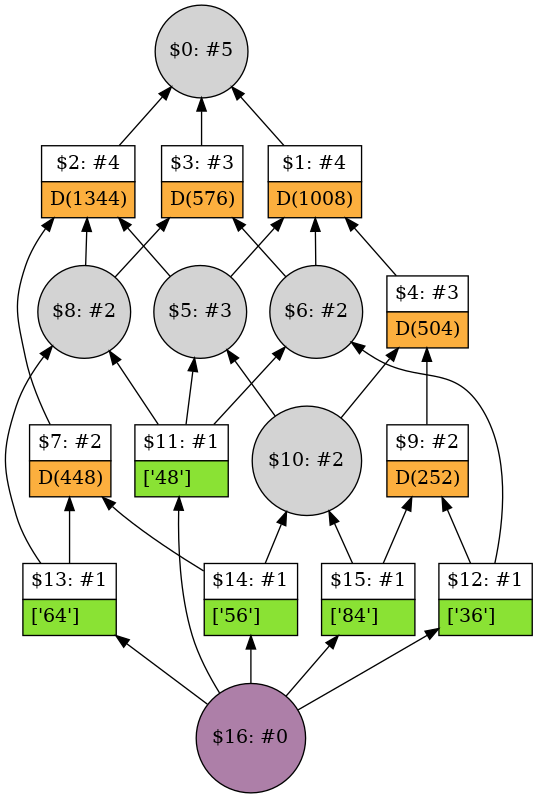
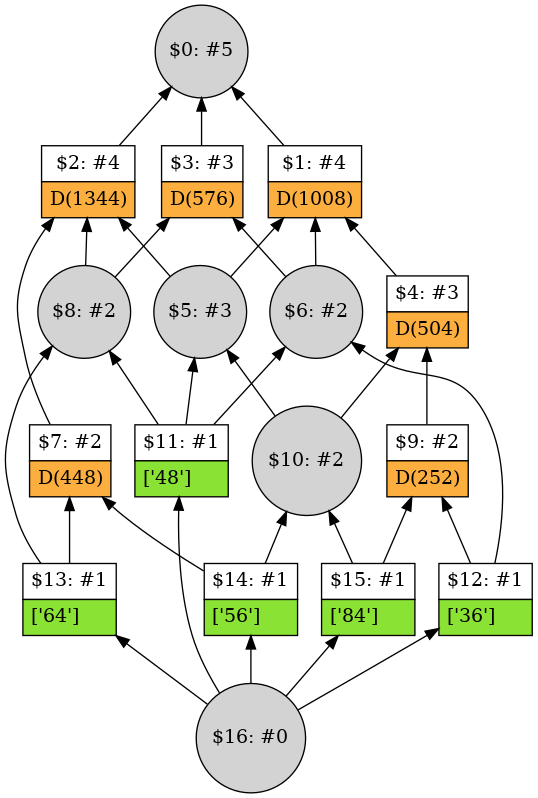
[11]:
lattice.apply([MultipleStrategy(characteristic)])
HasseDiagram(
lattice,
graph_renderer=ConceptLatticeRenderer(),
domain_renderer=ConceptRenderer(),
edge_renderer=HierarchicalRenderer(),
)
[11]:
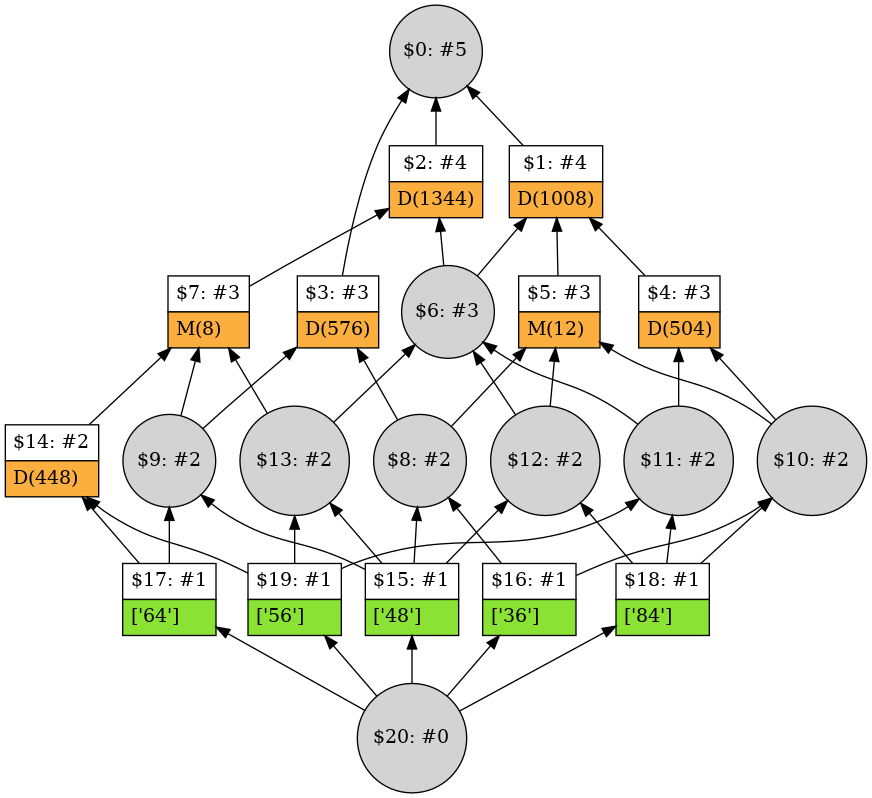
[12]:
from galactic.concepts import ConceptTable
ConceptTable(lattice)
[12]:
Concept |
Individuals |
Predicates |
---|---|---|
0 |
|
|
1 |
|
|
2 |
|
|
3 |
|
|
4 |
|
|
5 |
|
|
6 |
|
|
7 |
|
|
8 |
|
|
9 |
|
|
10 |
|
|
11 |
|
|
14 |
|
|
15 |
|
|
16 |
|
|
17 |
|
|
18 |
|
|
19 |
|
|
20 |
|
[13]:
ConceptTable(lattice, all_individuals=True, all_predicates=True)
[13]:
Concept |
Individuals |
Predicates |
---|---|---|
0 |
|
|
1 |
|
|
2 |
|
|
3 |
|
|
4 |
|
|
5 |
|
|
6 |
|
|
7 |
|
|
8 |
|
|
9 |
|
|
10 |
|
|
11 |
|
|
12 |
|
|
13 |
|
|
14 |
|
|
15 |
|
|
16 |
|
|
17 |
|
|
18 |
|
|
19 |
|
|
20 |
|
[14]:
display(*lattice.domain)
Predicates:
M(4)
D(4032)
Individuals:
48
36
64
56
84
Predicates:
M(4)
D(1008)
Individuals:
48
36
56
84
Predicates:
M(4)
D(1344)
Individuals:
48
64
56
84
Predicates:
M(4)
D(576)
Individuals:
48
36
64
Predicates:
M(4)
D(504)
Individuals:
36
56
84
Predicates:
M(12)
D(1008)
Individuals:
48
36
84
Predicates:
M(4)
D(336)
Individuals:
48
56
84
Predicates:
M(8)
D(1344)
Individuals:
48
64
56
Predicates:
M(12)
D(144)
Individuals:
48
36
Predicates:
M(16)
D(192)
Individuals:
48
64
Predicates:
M(12)
D(252)
Individuals:
36
84
Predicates:
M(28)
D(168)
Individuals:
56
84
Predicates:
M(12)
D(336)
Individuals:
48
84
Predicates:
M(8)
D(336)
Individuals:
48
56
Predicates:
M(8)
D(448)
Individuals:
64
56
Predicates:
M(48)
D(48)
Individuals:
48
Predicates:
M(36)
D(36)
Individuals:
36
Predicates:
M(64)
D(64)
Individuals:
64
Predicates:
M(84)
D(84)
Individuals:
84
Predicates:
M(56)
D(56)
Individuals:
56
Predicates:
M(0)
D(1)
Individuals:
Reduced context
[15]:
from galactic.algebras.lattice import ReducedContextDiagram
ReducedContextDiagram(
lattice,
domain_renderer=ConceptRenderer(join_irreducible=True),
co_domain_renderer=ConceptRenderer(meet_irreducible=True),
)
[15]:
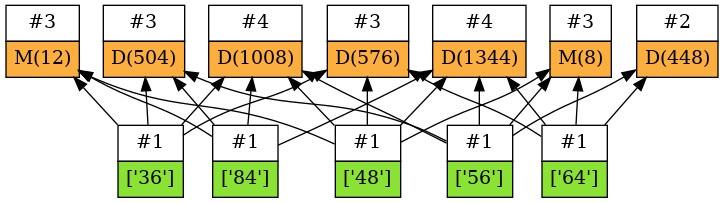
[16]:
from galactic.algebras.relational import BinaryTable
BinaryTable(
lattice.reduced_context,
domain_renderer=ConceptRenderer(join_irreducible=True),
co_domain_renderer=ConceptRenderer(meet_irreducible=True),
)
[16]:
@0 |
@1 |
@2 |
@3 |
@4 |
@5 |
@6 |
|
---|---|---|---|---|---|---|---|
84 |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
|||
56 |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
||
36 |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
|||
48 |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
||
64 |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |