Concept lattice¶
A population can be created using a collection of python objects:
[1]:
from galactic.population import Population
individuals = {48: 48, 36: 36, 64: 64, 56:56, 84: 84}
population=Population(individuals)
population
[1]:
<galactic.population.Population at 0x7f88cc4b7550>
[2]:
list(population)
[2]:
['48', '36', '64', '56', '84']
A lattice can be created from a population using a set of strategies:
[3]:
from galactic.algebras.poset.collections import HasseDiagram
from galactic.concepts.lattice import Lattice
from galactic.characteristics import identity
from galactic.examples.arithmetic.strategies import \
MultipleStrategy, DivisorStrategy
lattice = Lattice(
population=population,
strategies=[
MultipleStrategy(identity),
DivisorStrategy(identity),
]
)
[4]:
list(str(concept) for concept in lattice)
[4]:
['M(4) and D(4032)',
'M(4) and D(1008)',
'M(4) and D(1344)',
'M(4) and D(576)',
'M(12) and D(1008)',
'M(4) and D(504)',
'M(4) and D(336)',
'M(8) and D(1344)',
'M(12) and D(144)',
'M(16) and D(192)',
'M(12) and D(252)',
'M(12) and D(336)',
'M(28) and D(168)',
'M(8) and D(336)',
'M(8) and D(448)',
'M(48) and D(48)',
'M(36) and D(36)',
'M(64) and D(64)',
'M(84) and D(84)',
'M(56) and D(56)',
'M(0) and D(1)']
[5]:
HasseDiagram(lattice)
[5]:
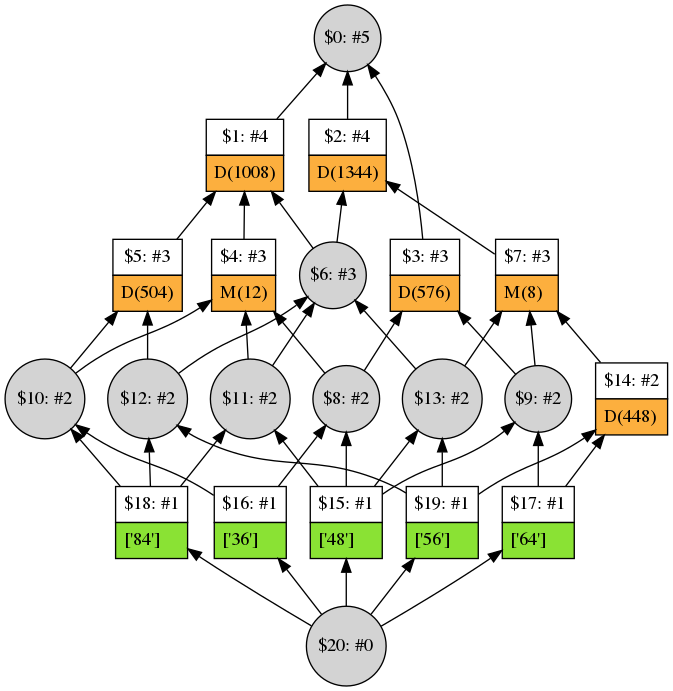
[6]:
HasseDiagram(lattice,show_predicates=True)
[6]:
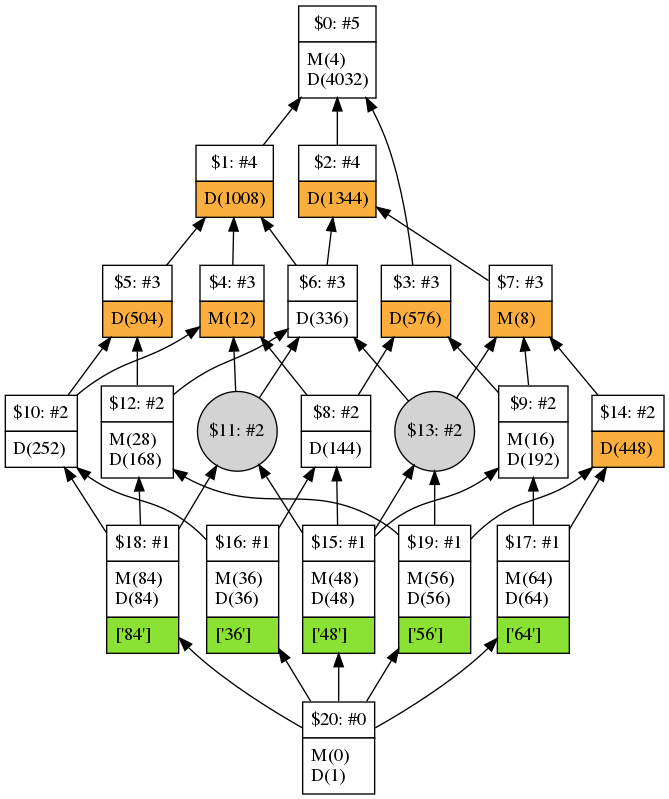
[7]:
from galactic.concepts.lattice import Table
Table(lattice)
[7]:
Concept |
Individuals |
Predicates |
---|---|---|
0 |
|
|
1 |
|
|
2 |
|
|
3 |
|
|
4 |
|
|
5 |
|
|
6 |
|
|
7 |
|
|
8 |
|
|
9 |
|
|
10 |
|
|
12 |
|
|
14 |
|
|
15 |
|
|
16 |
|
|
17 |
|
|
18 |
|
|
19 |
|
|
20 |
|
[8]:
Table(lattice,all_individuals=True, all_predicates=True)
[8]:
Concept |
Individuals |
Predicates |
---|---|---|
0 |
|
|
1 |
|
|
2 |
|
|
3 |
|
|
4 |
|
|
5 |
|
|
6 |
|
|
7 |
|
|
8 |
|
|
9 |
|
|
10 |
|
|
11 |
|
|
12 |
|
|
13 |
|
|
14 |
|
|
15 |
|
|
16 |
|
|
17 |
|
|
18 |
|
|
19 |
|
|
20 |
|
[9]:
display(*lattice)
Predicates:
M(4)
D(4032)
Individuals:
48
36
64
56
84
Predicates:
M(4)
D(1008)
Individuals:
48
36
56
84
Predicates:
M(4)
D(1344)
Individuals:
48
64
56
84
Predicates:
M(4)
D(576)
Individuals:
48
36
64
Predicates:
M(12)
D(1008)
Individuals:
48
36
84
Predicates:
M(4)
D(504)
Individuals:
36
56
84
Predicates:
M(4)
D(336)
Individuals:
48
56
84
Predicates:
M(8)
D(1344)
Individuals:
48
64
56
Predicates:
M(12)
D(144)
Individuals:
48
36
Predicates:
M(16)
D(192)
Individuals:
48
64
Predicates:
M(12)
D(252)
Individuals:
36
84
Predicates:
M(12)
D(336)
Individuals:
48
84
Predicates:
M(28)
D(168)
Individuals:
56
84
Predicates:
M(8)
D(336)
Individuals:
48
56
Predicates:
M(8)
D(448)
Individuals:
64
56
Predicates:
M(48)
D(48)
Individuals:
48
Predicates:
M(36)
D(36)
Individuals:
36
Predicates:
M(64)
D(64)
Individuals:
64
Predicates:
M(84)
D(84)
Individuals:
84
Predicates:
M(56)
D(56)
Individuals:
56
Predicates:
M(0)
D(1)
Individuals:
Reduced context¶
[10]:
from galactic.algebras.lattice.contexts import ReducedContext
[11]:
context = ReducedContext(lattice)
[12]:
context
[12]:
$1 |
$2 |
$3 |
$4 |
$5 |
$7 |
$14 |
|
---|---|---|---|---|---|---|---|
64 |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
|||
48 |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
||
36 |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
|||
84 |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
|||
56 |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |
\(\checkmark\) |